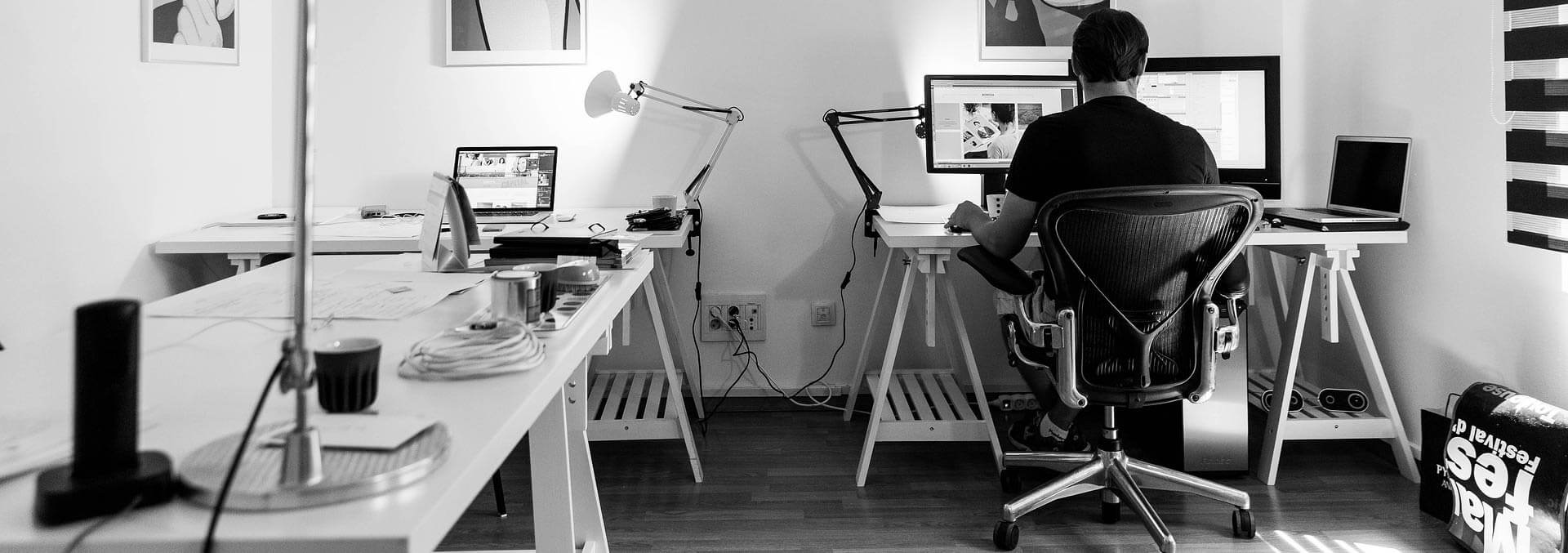
JSON to TSV
JSON (JavaScript Object Notation) and TSV (Tab-Separated Values) are two different formats for representing structured data. Converting JSON to TSV involves parsing the JSON data and extracting the relevant fields to create a tab-separated table.
Here's a basic example of how you might convert JSON to TSV using Python:
import json
import csv
# Sample JSON data
json_data = '''
[
{"name": "John", "age": 30, "city": "New York"},
{"name": "Alice", "age": 25, "city": "Los Angeles"},
{"name": "Bob", "age": 35, "city": "Chicago"}
]
'''
# Parse JSON
data = json.loads(json_data)
# Extract headers and rows
headers = list(data[0].keys())
rows = [list(entry.values()) for entry in data]
# Write to TSV file
with open('data.tsv', 'w', newline='') as tsvfile:
writer = csv.writer(tsvfile, delimiter='\t')
writer.writerow(headers)
writer.writerows(rows)
In this example:
- We first parse the JSON data using
json.loads()
to convert it into a Python data structure. - Then, we extract the keys of the first object in the JSON data to use them as column headers.
- We create a list of lists where each inner list represents a row of data.
- Finally, we write the headers and rows into a TSV file using the
csv.writer
module, specifying the tab ('\t') as the delimiter.
This will create a file named data.tsv
with the following content:
name age city
John 30 New York
Alice 25 Los Angeles
Bob 35 Chicago
You can adjust this code according to the structure of your JSON data and your specific requirements.